[Task 3] Basic Powershell Commands
Let’s explore before answering the questions
Get-Help Get-Command
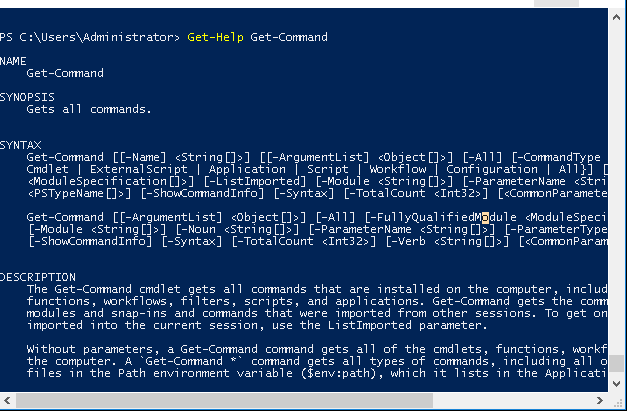
Get-Help Get-Command -Examples
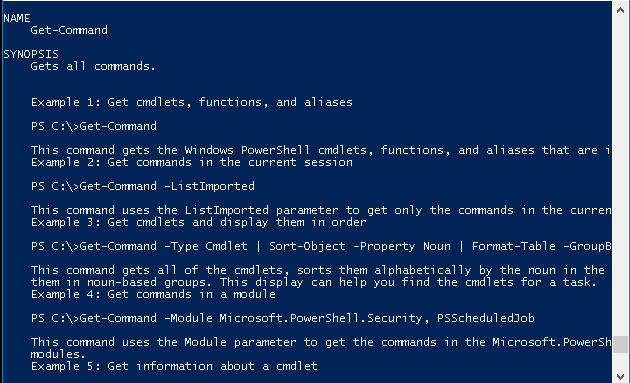
Get-Command New-*

Get-Member

Get-Command | Get-Member -MemberType Method

Get-ChildItem

Get-ChildItem | Select-Object -Property Mode,Name

Get-Service | Where-Object -Property Status -eq Stopped
- What is the location of the file “interesting-file.txt”
I guess that It has something to do with “Get-ChildItem”, but I need to know what paremeters I must use.
After researching in https://sid-500.com/2017/07/25/powershell-cmdlets-list-all-availabe-parameters-without-using-the-help/
List parameters
(Get-Command Get-ChildItem).Parameter
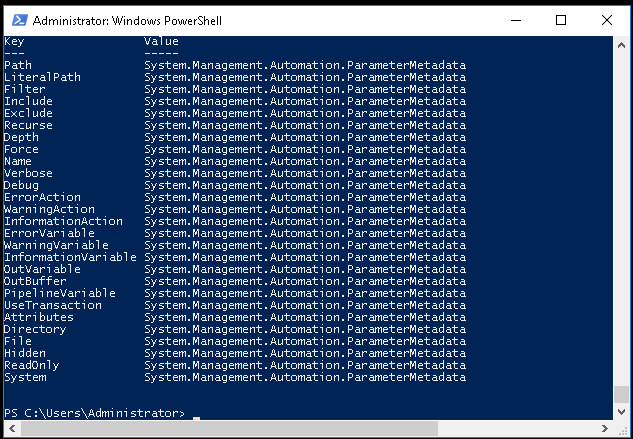
Get-ChildItem -Path C:/ -Name interesting-file.txt -Recurse -File
It’s not working.

Use “-Include” instead
Get-ChildItem -Path C:/ -Include interesting-file.txt -Recurse -File
It’s not working with error.
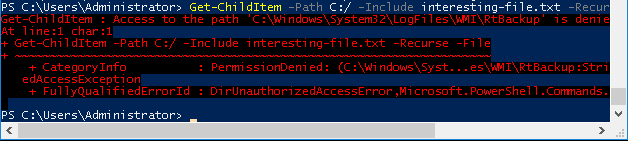
Let’s ignore error
Get-ChildItem -Path C:\ -Include interesting-file.txt -File -Recurse -ErrorAction SilentlyContinue
It’s not working with error.

Get-ChildItem -Path C:\ -Include *interesting-file.txt* -File -Recurse -ErrorAction SilentlyContinue
It’s work.
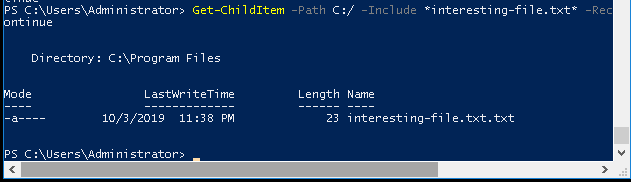
2. Specify the contents of this file
Get-Content "C:\Program Files\interesting-file.txt.txt"

3. How many cmdlets are installed on the system(only cmdlets, not functions and aliases)?
At first, I use this command:
Get-Command | measure

But the answer is wrong. So I have to see the whole result first
Get-Command
There’s a “Cmdlet”, but I don’t know the parameter name.

Let’s get parameter name first.
Get-Command | Select-Object -First 1
The parameter is “CommandType”

Get-Command | Where-Object -Parameter CommandType -eq Cmdlet | measure
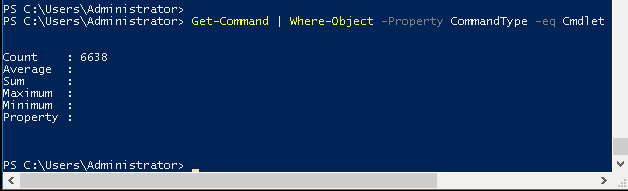
4. Get the MD5 hash of interesting-file.txt
Get-Command *hash*
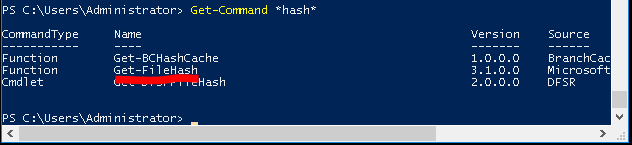
Get-FileHash
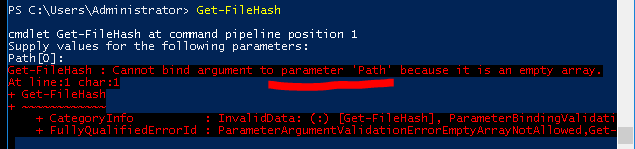
Let’s list the parameters
(Get-Command Get-FileHash).Parameters
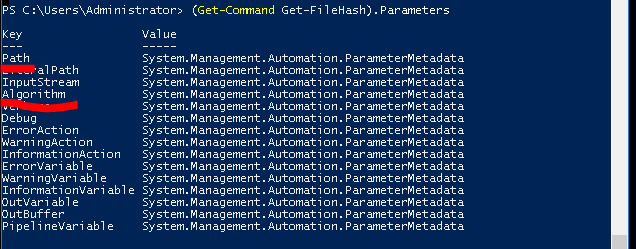
Get-FileHash -Path "C:\Program Files\interesting-file.txt.txt" -Algorithm MD5
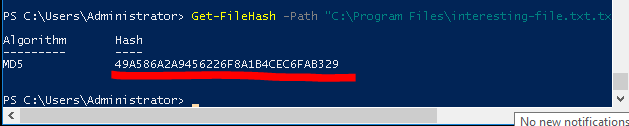
5. What is the command to get the current working directory?
Get-Location

6. Does the path “C:\Users\Administrator\Documents\Passwords” Exist(Y/N)?
Get-Location -Path "C:\Users\Administrator\Documents\Passwords"
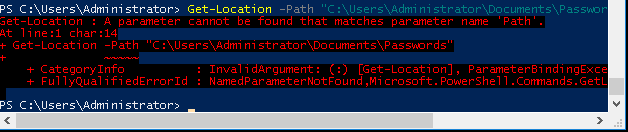
7. What command would you use to make a request to a web server?
Reference: https://docs.microsoft.com/en-us/powershell/module/microsoft.powershell.utility/invoke-webrequest?view=powershell-7
Invoke-WebRequest

8. Base64 decode the file b64.txt on Windows.
Search file first
Get-ChildItem -Path C:/ -Include *b64.txt* -Recurse -File
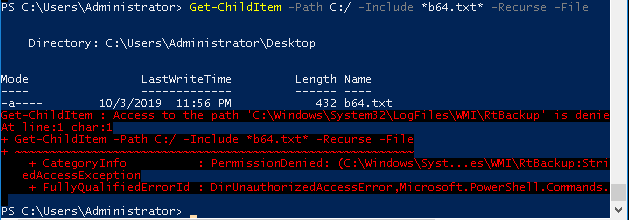
C:\Users\Administrator\Desktop\b64.txt
Use CertUtil
certutil -decode "C:\Users\Administrator\Desktop\b64.txt" out.txt
Read file
Get-Content out.txt

[Task 4] Enumeration
- How many users are there on the machine?
Get-LocalUser

2. Which local user does this SID(S-1–5–21–1394777289–3961777894–1791813945–501) belong to?
(Get-Command Get-LocalUser).Parameters
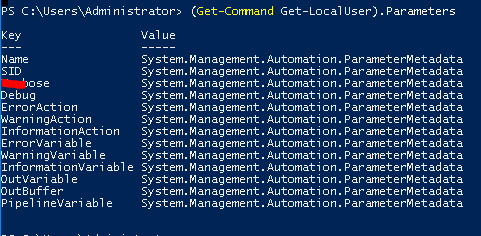
Get-LocalUser -SID "S-1-5-21-1394777289-3961777894-1791813945-501"

3. How many users have their password required values set to False?
List property
Get-LocalUser | Get-Member

Get-LocalUser | Where-Object -Property PasswordRequired -Match false

4. How many local groups exist?
Get-LocalGroup | measure
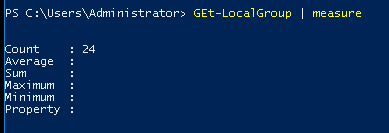
5. What command did you use to get the IP address info?
Get-NetIPAddress
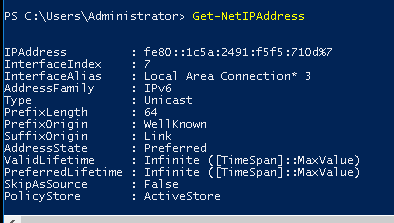
6. How many ports are listed as listening?
Get-NetTCPConnection
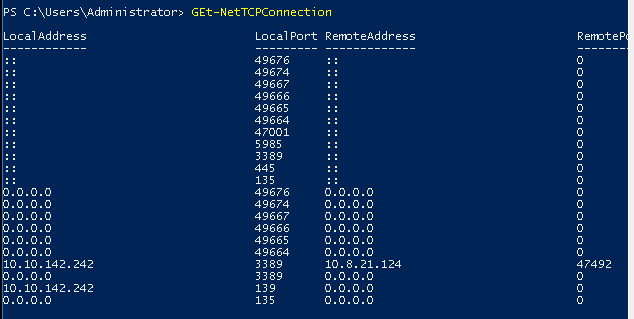
Get-NetTCPConnection | Get-Member
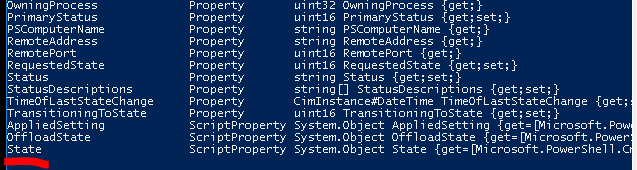
GEt-NetTCPConnection | Format-List -Property State
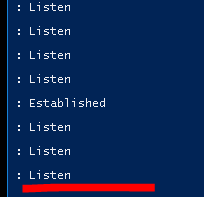
GEt-NetTCPConnection | Where-Object -Property State -Match Listen

GEt-NetTCPConnection | Where-Object -Property State -Match Listen | measure

7. What is the remote address of the local port listening on port 445?
ANS: ::
8. How many patches have been applied?
Get-Hotfix
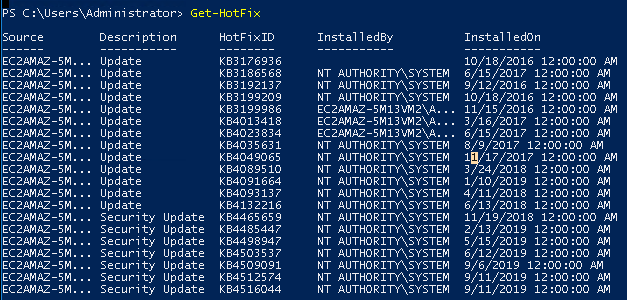
Get-Hotfix | measure
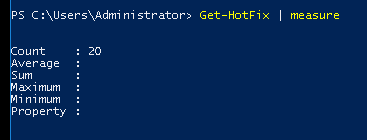
9. When was the patch with ID KB4023834 installed?
(Get-Command Get-HotFix).Parameters

Get-Hotfix -Id KB4023834

10. Find the contents of a backup file.
Get-ChildItem -Path C:\ -Include *.bak* -File -Recurse -ErrorAction SilentlyContinue
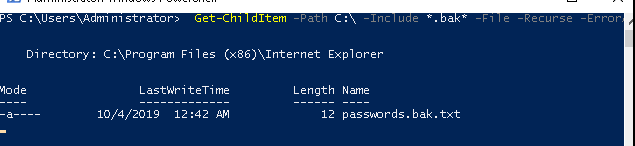
Path -> C:\Program Files (x86)\Internet Explorer\passwords.bak.txtGet-Content "C:\Program Files (x86)\Internet Explorer\passwords.bak.txt"

11. Search for all files containing API_KEY
Get-ChildItem C:\* -Recurse | Select-String -pattern API_KEY
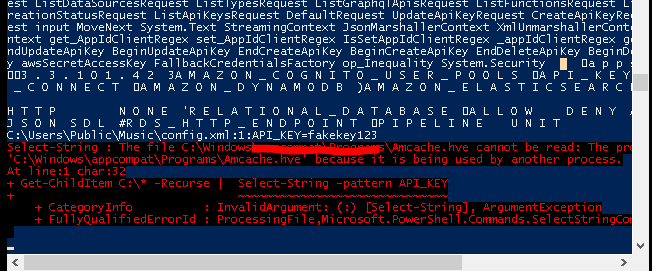
12. What command do you do to list all the running processes?
Get-Process
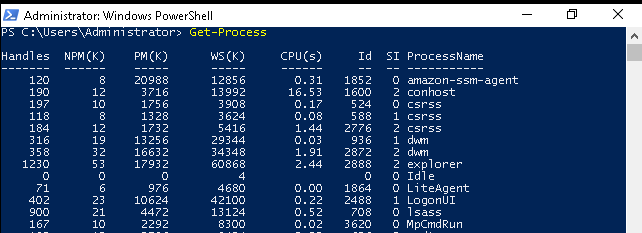
13. What is the path of the scheduled task called new-sched-task?
Get-ScheduleTask
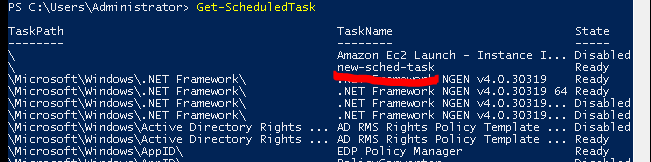
Get-ScheduleTask -TaskName new-sched-task

14. Who is the owner of the C:\
Get-Acl c:/
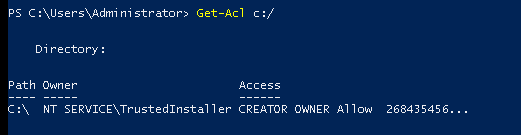
[Task 5] Basic Scripting Challenge
- What file contains the password?
Let’s try command first
Get-ChildItem -Path "C:\Users\Administrator\Desktop\emails\*" -Recurse | Select-String -Pattern password

Open Windows PowerShell ISE

Write the script
$path = "C:\Users\Administrator\Desktop\emails\*"
$string_pattern = "password"
$command = Get-ChildItem -Path $path -Recurse | Select-String -Pattern $String_patternecho $command
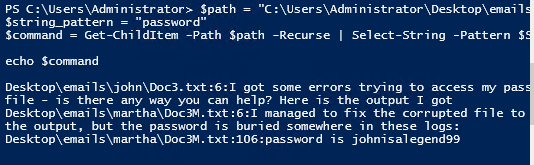
2. What is the password?
ANS: It’s in #1.
3. What files contains an HTTPS link?
$path = "C:\Users\Administrator\Desktop\emails\*"
$string_pattern = "https://"
$command = Get-ChildItem -Path $path -Recurse | Select-String -Pattern $String_patternecho $command
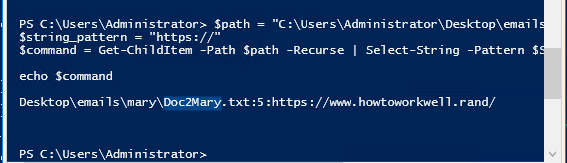
[Task 6] Intermediate Scripting
- How many open ports did you find between 130 and 140(inclusive of those two)?
I’m not sure if it’s correct
for($i=130; $i -le 140; $i++){
Test-NetConnection localhost -Port $i
}
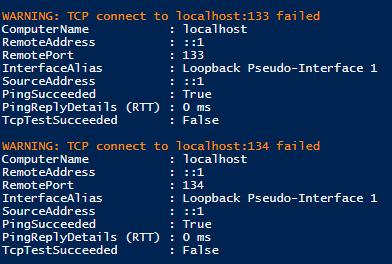
At first, I answered “1”, but it’s not correct.
So, I answered “11”.